Change the color for each player
Hello, I'm a new to Normcore. Forgive me for being a newbie question.
I tried the official website Multiplayer Drawing. I would like to change the color of the drawing for each player, as shown in the first video.
*I was able to synchronize and change the colors and materials of all players.
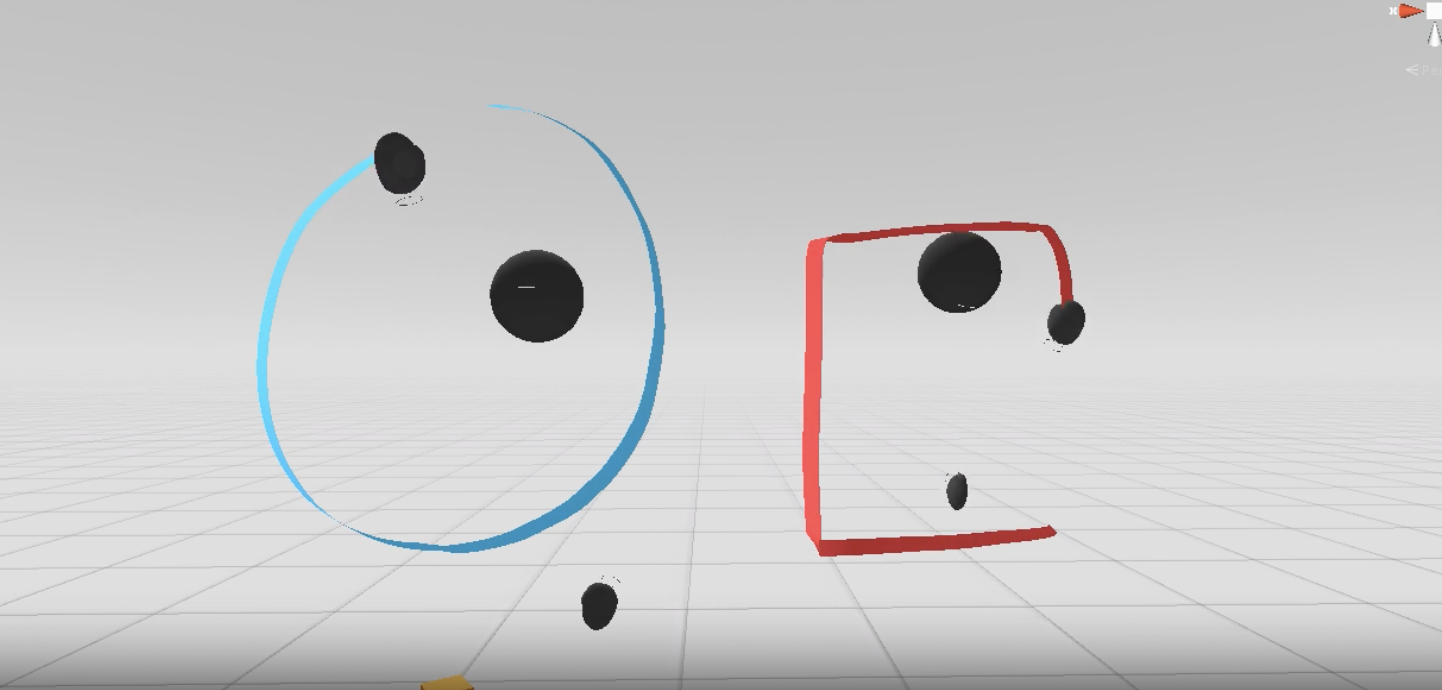
4 Replies
Hi @Naopyon. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
To change the color for each player in a Normcore multiplayer drawing app, you'll need to modify the synchronization of custom data. While I don't have specific information about the Multiplayer Drawing example you're referring to, I can provide some guidance based on Normcore's custom data synchronization principles.
Here's a general approach you could take:
1. Create a custom
RealtimeComponent
for player color, similar to the ColorSync
example in the documentation.
2. In your player object or brush object, add this custom component to synchronize the color for each player.
3. Modify your drawing logic to use the color from this component when drawing.
Here's an example of how you might create a PlayerColorSync
component:
You would also need to create a corresponding PlayerColorSyncModel
:
Remember to compile the model after creating it, as described in the Generating model code documentation (https://normcore.io/documentation/realtime/synchronizing-custom-data#generating-model-code-in-unity-20192020).
To use this in your drawing app, you would:
1. Add the PlayerColorSync
component to each player object.
2. When drawing, use playerColorSync.GetColor()
to get the current player's color.
3. If you want to change a player's color, call playerColorSync.SetColor(newColor)
.
This approach should allow each player to have their own synchronized color. However, please note that this is a general solution and you might need to adapt it to fit the specific structure of the Multiplayer Drawing example you're working with.
For more detailed information on synchronizing custom data in Normcore, you can refer to the Synchronizing Custom Data documentation (https://normcore.io/documentation/realtime/synchronizing-custom-data#creating-a-custom-realtime-component).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------@Normcore Assistant mmm...yes, I was able to synchronize and change the color using RealtimeComponent. Shoud I add two player objects or two brush object in the scene and attach the script to change for Each player?
Let me check the knowledge base for you again. One moment! :books:
----------------------
If you want each player to have their own color, you should add the
PlayerColorSync
component to the player object. This way, each player will have their own instance of the PlayerColorSync
component, and therefore their own color.
Here's a step-by-step guide:
1. Add the PlayerColorSync
component to your player prefab. This prefab should be the one you're using with Realtime.Instantiate
to create a new player when someone joins the game.
2. In your drawing logic, get the player's color by accessing the PlayerColorSync
component on the player object. For example, if player
is a reference to the player object, you could use player.GetComponent<PlayerColorSync>().GetColor()
to get the player's color.
3. Use this color when drawing. The exact way to do this will depend on how your drawing logic is implemented.
Remember, the PlayerColorSync
component should be added to the player object, not the brush object. This is because the player object is what gets instantiated for each player in the game, so each player will have their own PlayerColorSync
component with their own color.
If you want to change a player's color, you can call SetColor(newColor)
on their PlayerColorSync
component. For example, player.GetComponent<PlayerColorSync>().SetColor(newColor)
.
This approach should allow each player to have their own color that is used when they draw. However, please note that this is a general solution and you might need to adapt it to fit the specific structure of your game.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@924354492781170788> Kapa.ai is still learning and improving, please let me know how I did by reacting below.