Networked Object Pooling Questions
Hello! I'm new to Normcore, & I'm trying to get my bearings in terms of adapting our current Object Pooling system for Normcore. I'll introduce the problem and my primary questions, and attach my current script.
I've created a script called RealtimeObjectPool which implements the interface IRealtimePrefabInstantiateDelegate. I have a few questions, some about design and some more technical.
1. I have a CreateObjectPool() function that runs when a room is created. This creates the object pool on all clients independently.
-- The first problem, or question, I have is... I'm assuming I should use the Realtime.Instantiate() to spawn the pool objects right? Given that they are prefabs with realtime views, and the console gets mad at me if I use the regular Unity Instantiate(). But I'm unsure how to smoothly handle that. Should I have the Host only call Realtime.Instantiate() in CreateObjectPool() instead? But if so, how do I hook the spawned list of pooled objects to the client? Need some guidance on best practice there.
2. Outside of the pool, if I have a manager request a pooled object, should I do a call to Pool.Instance.InstantiateRealtimePrefab()? Or is it the case that, since the delegate is implemented in a script on the same Object as the realtime component, I simply need to call Realtime.Instantiate() from a manager and Realtime will automatically use my custom implementation instead of the default spawning?
Overall, I think I would really appreciate a Recipe for Networked Object Pooling since there's nothing that shows an end to end simple implementation (Addressables helped a little, but not too much, and the IRealtimePrefabInstantiateDelegate documentation is pretty sparse). For now I would just like a little bit of clarity / advice!
I've attached my code as a picture since not enough characters remaining. Thanks in advance!!
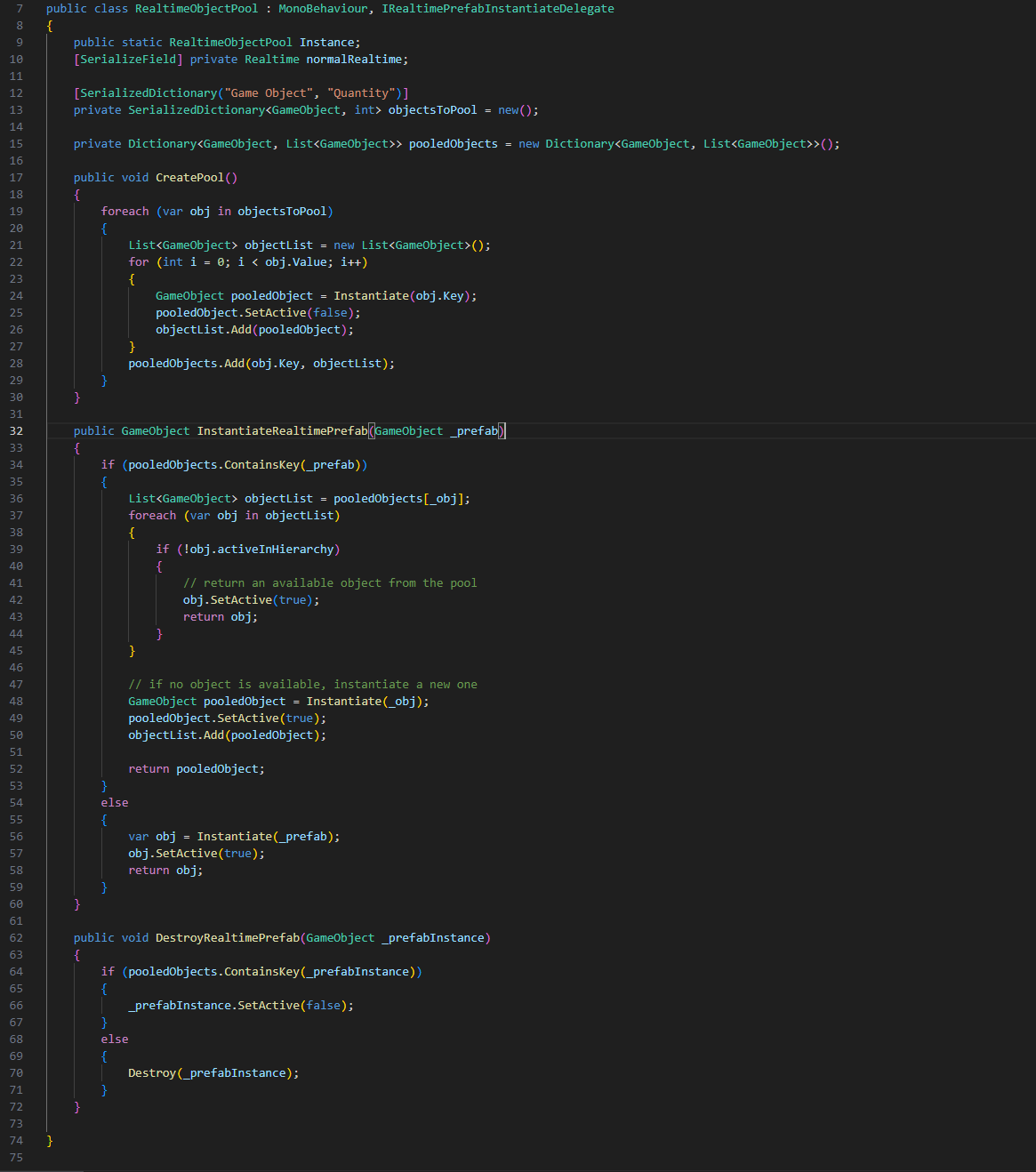
7 Replies
Hi @KingScroll. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
Thank you for your questions about implementing object pooling with Normcore. I'll address your main points based on the available information from Normcore's documentation.
1. Regarding creating the object pool:
You're correct that you should use
Realtime.Instantiate()
for spawning prefabs with RealtimeViews. However, for object pooling, you'll want to handle this differently. Instead of creating the pool on all clients independently, you should have the host create the pool and then synchronize it across all clients.
The IRealtimePrefabInstantiateDelegate interface is designed to help with this. You can implement this interface to customize how prefabs are instantiated and destroyed. Here's a suggested approach:
- Have the host create the object pool using Realtime.Instantiate()
.
- In your InstantiateRealtimePrefab()
method, instead of creating a new object, return an object from your pool.
- In your DestroyRealtimePrefab()
method, return the object to the pool instead of destroying it.
2. Regarding requesting a pooled object:
You should call Realtime.Instantiate()
from your manager. Normcore will automatically use your custom implementation of IRealtimePrefabInstantiateDelegate
if it's attached to the same GameObject as the Realtime component.
Here's a basic example of how you might implement this:
Attach this script to the same GameObject as your Realtime component.
Unfortunately, there isn't a specific "Recipe for Networked Object Pooling" in the Normcore documentation. The closest example is the Using Addressables guide, which demonstrates how to customize prefab loading and instantiation.
For a more complete implementation, you might want to consider:
- Initializing the pool with a certain number of objects when the room is created.
- Implementing a method to grow the pool if needed.
- Handling ownership and lifetime flags appropriately for pooled objects.
Remember to always use Realtime.Instantiate()
and Realtime.Destroy()
for networked objects, even when working with the pool.
I hope this helps clarify the approach to networked object pooling with Normcore. If you need more specific guidance, I recommend reaching out to the Normcore community on their Discord for further assistance.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@144914135245651968> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
@NormalMark definitely worth us putting together a pooling sample for the docs
For #2 you call Realtime.Instantiate() on one client, it instructs all clients (including the local one) to instantiate that prefab by name, and all clients will locally call your prefab delegate. The way you connect it to Realtime is by having the monobehaviour you've posted here live on the same game object as Realtime. There's no concept of a host with this pooling architecture. The prefab is instantiated in the room and stored in the datastore as a model, all clients locally instantiate a prefab to act as the view for that model, and they'll all consult their local instance of your object pool class that you've made here.
For #3 (also labelled as #2) the latter is correct. You don't need to call anything on the pool directly yourself. Realtime will see the MonoBehaviour on the same game object that implements the two realtime prefab delegate interfaces and will call the methods you've implemented here.
Perfect! I appreciate the clarification
Ahh, I see now. That makes sense. This is very helpful! Thanks. I'll give it a go tomorrow and report back.
sounds good! keep me posted
Using Realtime.Instantiate() in my manager scripts did indeed work correctly!
Glad to hear it!