Problem syncing objects after instanciating.
I have an app that has a PC client and a VR client - using the Cube play and VR player prefabs from the samples with some changes i made
I have a task to spawn an object that would be a child of the VR head so it will move in reference to the VR Player.
However the spawning and manipulation of the object must happen on the PC client.
I'll attach the code in a comment.
I see that the object is created on the PC , and its parent is indeed the VRHead reference, but, i can't check it on the VR and it spwans on the ground instead of attached to the head.
Is the parenting thing wrong or what am i doingsomethign else wrong?
I see the object being swpawns in the VR just not moving with the head
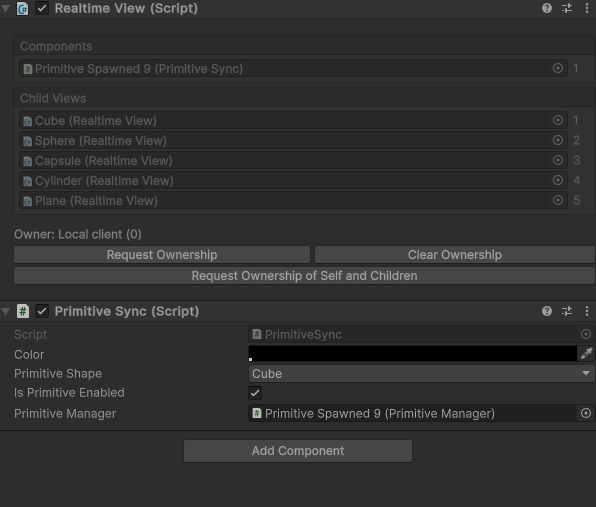
11 Replies
Hi @SoundGuy2. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
This is my code:
// local is a bool that determines if the object is local or a child of the VR player
// Instantiate the CubePlayer for this client once we've successfully connected to the room. Position it 1 meter in the air.
var options = new Realtime.InstantiateOptions {
ownedByClient = local, // Make sure the RealtimeView on this prefab is owned by this client.
preventOwnershipTakeover = local, // Prevent other clients from calling RequestOwnership() on the root RealtimeView.
useInstance = _realtimeUserInstance // Use the instance of Realtime that fired the didConnectToRoom event.
};
GameObject go = Realtime.Instantiate(PrimitivePrefab.name, options);
RealtimeTransform rtTransform = go.GetComponent<RealtimeTransform>();
if (rtTransform == null) {
Debug.LogError("No RealtimeTransform in Primitive Spawner");
}
if (local) {
go.transform.parent = this.transform;
}
else {
_vrHead ??= FindFirstObjectByType<VRHead>();
if (_vrHead) {
go.transform.parent = _vrHead.transform;
} else {
Debug.LogError("Missing VRHead to spawn attached primitive");
}
}
go.GameObject().transform.localPosition = pos; go.GameObject().transform.localRotation = rot;
go.GameObject().transform.localPosition = pos; go.GameObject().transform.localRotation = rot;
----------------------
Based on the information provided in the knowledge sources, I can offer some insights into your problem with syncing objects after instantiation in Normcore. However, it's important to note that the knowledge sources don't contain specific information about parenting objects to VR players or manipulating objects from a PC client. Nevertheless, I can provide some general guidance based on Normcore's behavior.
1. Instantiation: Make sure you're using
Realtime.Instantiate()
instead of Unity's Instantiate()
to create your object. This ensures that the prefab is instantiated on all clients and that all RealtimeComponents are properly connected. Here's an example:
Room > Server Authority + Ownership + Lifetime Flags
2. Parenting: Normcore doesn't automatically synchronize parent relationships between objects. This could explain why your object appears on the ground in VR instead of being attached to the head.
Realtime > RealtimeTransform > Extra notes states:
"Don't reparent game objects with RealtimeTransform on them! RealtimeTransform does not track the parent of a RealtimeTransform and cannot synchronize it at runtime."
Instead of parenting, they suggest creating an empty game object on your player character at the pick-up point and using a script to synchronize the world position of the pick-up point object and the RealtimeTransform object you want to pick up.
3. Synchronization: Ensure that your spawned object has a RealtimeView and necessary RealtimeComponents (like RealtimeTransform) to synchronize its position and rotation across clients.
4. Ownership: Since you're manipulating the object from the PC client, make sure the PC client has ownership of the object. You can set this when instantiating:
5. Timing: If you're dealing with RealtimeViews in a scene file, you need to wait until realtime.connected == true
, or the didConnectToRoom
event has fired before interacting with them.
Realtime > Common Questions
To solve your specific issue, you might need to:
1. Use Realtime.Instantiate() to create your object.
2. Instead of parenting, create a script that synchronizes the world position and rotation of your object with the VR player's head position.
3. Ensure your object has the necessary RealtimeComponents for synchronization.
4. Make sure the PC client has ownership of the object for manipulation.
If these suggestions don't solve your problem, you might need to provide more details about your specific implementation or consult the Normcore documentation for more advanced synchronization techniques.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1081953198014734357> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
is there no way to reparent realtime objects?
Unfortunately Unity doesn’t give us a reliable way to track parent/child relationships
how do i sync a realtime transform world coordicates instead of local ones?
The only case where RT will sync world coordinates is if a rigidbody is present. In general we recommend using RT to sync all parent game objects.
yeah but that's the problem - the VR head is synced between clients but i can't seen to be able to set the new spanwed object to be under the VR head as aparent. i'll try to think of a workdaround...
Create a component that syncs the world space position of the head to the secondary object. And keep the secondary object in world space so that way the world and local transform positions are the same