Manually syncing position, rotation at 20hz?
If I were manually syncing an object position and rotation, should the model setter just be setting every frame or can resources be saved by setting it only 20x a second becuase thats what normcore would use anyway? It woudnlt' be in sync with normcores refresh anyway i'd assume so how does that work? Is there a way to sync at normcores refresh rate?
Solution:Jump to solution
```cs
protected override void OnRealtimeModelReplaced(YourModel previousModel, YourModel currentModel) {
model.onWillWrite += OnModelWillWrite;
}
...
22 Replies
Hi @cryptomax. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
Normcore synchronizes changes to the server at a rate of 20Hz, meaning it sends the latest state every 50ms. If you change something and then change it back immediately, nothing will be sent to the server. Therefore, if you're manually syncing an object's position and rotation, you could potentially save resources by setting it only 20 times a second to align with Normcore's synchronization rate. However, it's important to note that this might not always result in the desired behavior, especially if the object is moving rapidly or if precise synchronization is required.
To synchronize an object's position and rotation with Normcore's refresh rate, you would typically use the
RealtimeTransform
component. This component automatically synchronizes the position, rotation, and scale of a GameObject. If the RealtimeTransform
component is owned locally, it will synchronize these properties to the datastore. If it's not owned locally, it will treat the values in the datastore as the source of truth and synchronize them to the object.
Here's how you can use the RealtimeTransform
component to synchronize an object:
1. Add a Realtime
component to an empty game object in your scene and configure the appKey so it can connect.
2. Add a RealtimeTransform
component to the object you want to synchronize.
3. If you want to move the object, you need to request ownership of it. You can do this by clicking the "Request Ownership" button on the RealtimeTransform
inspector.
Remember, if you're manually setting the position and rotation of an object and then immediately clearing ownership, nothing will happen because Normcore doesn't send changes to the server immediately when you change ownership or the value on a model. In this case, you should skip calling ClearOwnership()
. As long as the RealtimeView
's owner is None, then any other client in the future can just call RequestOwnership()
to take over the transform and move it.
For more information, you can refer to the following documentation:
- Synchronizing a GameObject (https://normcore.io/documentation/realtime#synchronizing-a-gameobject)
- RealtimeTransform (https://normcore.io/documentation/realtime/realtimetransform)
- Common Questions (https://normcore.io/documentation/realtime/common-questions#im-calling-requestownership-setting-the-position-of-a-transform-and-then-immediately-calling-clearownershipbut-nothing-happens-how-can-i-fix-this)
- Networked Physics (https://normcore.io/documentation/realtime/networked-physics#ownership--physics)
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@401986039964631060> kapa.ai is still learning and improving, please let us know how it did by reacting below
If you're using realtime transform I'd just update the position every frame and not worry about it, you won't be saving much performance and you can focus on bigger bottlenecks
I was trying to bypass using RT transform because they would have rigidbodies on them but be swapping in and out of kinematic and the majority of the time they would just be kinemic, so if there are 30-40 of these it could really add up to have the extra overhead over syncing two vector3s
but it seems like there is no particualr easy way to sync at normcores refresh rate
I believe you can hook into
model.onModelWillWrite
(or something like that) to get a callback for whenever a write will occur. Generally setting it via update is fine thoughlike this?
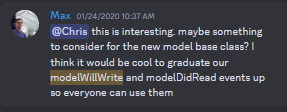
@Normcore Assistant do you ahve any info about modelWillWrite or modelDIdRead?
Let me check the knowledge base for you. One moment! :books:
----------------------
Yes,
modelWillWrite
and modelDidRead
are part of the RealtimeModelEvent
enum in Normcore. They are used to subscribe to specific events related to the serialization and deserialization of models. Here's a brief overview:
- OnWillWrite
: This event is dispatched before a serialization pass. Serialization is the process of converting the state of an object into a format that can be stored or transmitted and then reconstructed later.
- OnDidRead
: This event is dispatched after a deserialization pass. Deserialization is the reverse of serialization, it's the process of converting data from a stored or transmitted format back into an object.
These events are generally used with RealtimeCallback
.
Here's a brief description of each:
- OnWillWrite
: Dispatched before a serialization pass. This is when the state of the model is being prepared to be sent over the network.
- OnDidWrite
: Dispatched after a serialization pass. This is when the state of the model has been successfully prepared to be sent over the network.
- OnWillRead
: Dispatched before a deserialization pass. This is when the received data is about to be applied to the model.
- OnDidRead
: Dispatched after a deserialization pass. This is when the received data has been successfully applied to the model.
- OnDidReadProperties
: Dispatched after a model reads properties from a remote update. This is when the model has been updated with the latest state from the server.
For more information, you can refer to the RealtimeModelEvent documentation (https://normcore.io/documentation/reference/classes/Normal.Realtime.RealtimeModelEvent).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@401986039964631060> kapa.ai is still learning and improving, please let us know how it did by reacting below
@Normcore Assistant so could these be used to only send an update when it is going to write to the model? Do you have any example code?
Let me check the knowledge base for you. One moment! :books:
----------------------
Yes, you can use these events to control when updates are sent. Specifically, the
OnWillWrite
event can be used to determine when the model is about to be serialized and sent over the network. However, please note that Normcore handles the serialization and network transmission automatically, so you don't typically need to manually control when updates are sent.
Unfortunately, the provided knowledge sources do not include specific example code for using these events. However, you would generally use them with the RealtimeCallback
class to subscribe to these events and execute your own code when they occur.
Here's a general idea of how you might use these events:
Please note that this is a general example and might need to be adjusted based on your specific use case and the structure of your project.
For more information on how to use these events, please refer to the RealtimeModelEvent documentation (https://normcore.io/documentation/reference/classes/Normal.Realtime.RealtimeModelEvent).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@401986039964631060> kapa.ai is still learning and improving, please let us know how it did by reacting below
unsure if that is correct...
let me check my code
Solution
sweet so this should only fire when it would have updated saving like 2 thirds of the updates I would think?
potentially, granted stuff like this should usually be very light
copying over some small data
there are likely bigger perf bottlenecks elsewhere unless your set code is really expensive
might seem like splitting hairs but performance will be a problem on our new map so every single place i can save it will help
but yeah I get what you mean, I heavily optimized my main object network code
from all i can tell the main issues are just the quest 2 can only handle so many syncing rigidbodies
yeah that's checks out, gotta be careful with body updates
thanks!
ye np