Offline rig
how do I make a offline rig so when i join a server that rig is gone and now showd the player prefab thing
28 Replies
Hi @RatCatcherVr. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
It sounds like you're asking about how to handle a local offline rig that should be replaced by a networked player prefab when connecting to a Normcore room.
To achieve this, you can use Normcore's offline mode functionality and then switch to online mode when connecting to a server. Here's how you can approach this:
1. Create a local offline rig that's active by default in your scene
2. When connecting to a Normcore room, disable or destroy this offline rig
3. Use RealtimeAvatarManager to instantiate the networked player prefab
Here's a simple implementation approach:
For the networked player prefab, you'll want to use RealtimeAvatarManager which will automatically instantiate your avatar prefab when connected to a room. The RealtimeAvatarManager should be on the same GameObject as your Realtime component, and you'll need to set the localAvatarPrefab property to your player prefab. This prefab needs to include a RealtimeAvatar component on its root to function correctly.
When you connect to a room, the RealtimeAvatarManager will instantiate your player prefab and the offline rig will be disabled. When you disconnect, the player prefab will be destroyed and your offline rig will be re-enabled.
For more details on avatar management, check out the XR Avatars documentation.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
<@1245530684240695310> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
@Normcore Assistant how do i set it up?
Let me check the knowledge base for you again. One moment! :books:
----------------------
Based on our previous conversation about setting up an offline rig that gets replaced by a networked player prefab when connecting to a Normcore room, here's a step-by-step guide to set it up:
1. Create your offline rig:
- Add your VR camera rig/player controller to your scene
- This will be your local representation when not connected to a Normcore room
2. Add Normcore components:
- Add a GameObject to your scene
- Add a Realtime component to this GameObject
- Add a RealtimeAvatarManager component to the same GameObject
- Configure your App Key in the Realtime component
3. Create your networked avatar prefab:
- Create a prefab for your networked avatar
- Add a RealtimeView component to the root
- Add a RealtimeAvatar component to the root
- Place this prefab in a Resources folder
4. Configure the RealtimeAvatarManager:
- Set the "Local Avatar Prefab" field to your networked avatar prefab
5. Add the OfflineRigManager script:
- Add the script I shared previously to your scene
- Assign your offline rig to the "Offline Rig" field
- Assign your Realtime component to the "Realtime" field
When you connect to a room, the RealtimeAvatarManager will automatically instantiate your networked avatar prefab, and the OfflineRigManager will disable your offline rig. When you disconnect, your offline rig will be re-enabled.
For more detailed information about avatar setup, you can refer to the XR Avatars and Voice Chat guide which provides a complete walkthrough of setting up avatars in Normcore.
If you're using Meta avatars, Normcore also has a specific implementation for that which you can learn about in the Meta Avatars guide.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
<@1245530684240695310> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
@Normcore Assistant i do have the offline rig made
Let me check the knowledge base for you again. One moment! :books:
----------------------
Great! Since you already have your offline rig made, let's continue with the remaining steps to set up the switching between your offline rig and the networked player prefab:
1. Add Normcore components to your scene:
- Create an empty GameObject in your scene
- Add a Realtime component to this GameObject
- Add a RealtimeAvatarManager component to the same GameObject
- Configure your App Key in the Realtime component (you can get this from the dashboard)
2. Create your networked avatar prefab:
- Create a prefab that will represent players in the multiplayer room
- Add a RealtimeView component to the root of this prefab
- Add a RealtimeAvatar component to the root
- Place this prefab in a Resources folder in your project
3. Configure the RealtimeAvatarManager:
- In the inspector, set the "Local Avatar Prefab" field to reference your networked avatar prefab
4. Add the OfflineRigManager script to handle the switching:
5. Add this script to your scene:
- Add the OfflineRigManager script to a GameObject in your scene
- Assign your existing offline rig to the "Offline Rig" field
- Assign your Realtime component to the "Realtime" field
When you connect to a Normcore room, the RealtimeAvatarManager will automatically instantiate your networked avatar prefab, and the OfflineRigManager will disable your offline rig. When you disconnect, your offline rig will be re-enabled.
For more information on avatar management in Normcore, you can refer to the XR Avatars documentation.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
<@1245530684240695310> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
@Normcore Assistant ty
Let me check the knowledge base for you again. One moment! :books:
----------------------
You're welcome! I'm glad I could help you set up the switching between your offline rig and networked player prefab in Normcore. If you have any other questions about Normcore or need further assistance with your implementation, feel free to ask.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
<@1245530684240695310> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
you can connect in offline mode and just reuse your online assets
saves time
how?
@kapa.ai how do I connect to an offline room
Let me check the knowledge base for you again. One moment! :books:
----------------------
To connect to an offline room in Normcore, you can use the
offlineMode
parameter in the ConnectOptions
struct when calling the Connect()
method. This is useful for singleplayer games, tutorials, or experiences that don't require an internet connection.
Here's how to connect to an offline room:
Important things to know about offline mode:
1. Make sure to uncheck "Join Room on Start" on the Realtime component if you're manually calling Connect()
2. The clientID
will always return 0
in offline mode
3. The datastore state is cleared when you call Disconnect()
4. It's not possible to have two local clients connect to the same offline room
5. RealtimeComponents and scripts developed for online use will continue to function in offline mode without changes
This approach works well with your offline rig setup we discussed earlier - you can use offline mode for your local experience and then switch to online mode when you want to connect to a multiplayer room.
Offline Mode Documentation
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@162940911964258305> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
ty @Mechabit
@kapa.ai you dont have to initialize _realtime to anything in this code?
using Normal.Realtime;
using UnityEngine;
public class OfflineModeExample : MonoBehaviour {
[SerializeField]
private Realtime _realtime;
private void Start() { // Notify us when Realtime successfully connects to the room
_realtime.didConnectToRoom += DidConnectToRoom;
// Connect to "My Room" in offline mode
_realtime.Connect("My Room", new Room.ConnectOptions {
offlineMode = true
});
}
private void DidConnectToRoom(Realtime realtime) {
if (realtime.room.offlineMode) {
Debug.Log($"Connected to room in offline mode!");
}
}
}
private void Start() { // Notify us when Realtime successfully connects to the room
_realtime.didConnectToRoom += DidConnectToRoom;
// Connect to "My Room" in offline mode
_realtime.Connect("My Room", new Room.ConnectOptions {
offlineMode = true
});
}
private void DidConnectToRoom(Realtime realtime) {
if (realtime.room.offlineMode) {
Debug.Log($"Connected to room in offline mode!");
}
}
}
Let me check the knowledge base for you again. One moment! :books:
----------------------
You're right to question this! In the code example,
_realtime
is marked with [SerializeField]
but not initialized in the code itself. This is because:
The [SerializeField]
attribute exposes the private field in the Unity Inspector, allowing you to assign the Realtime component through the Unity Editor rather than in code.
Here's how you would set it up:
1. Add this script to a GameObject in your scene
2. In the Inspector, you'll see a field labeled "Realtime"
3. Drag and drop a GameObject that has a Realtime component onto this field, or use the object picker to select it
This is a common Unity pattern where references to components are assigned through the Inspector rather than programmatically.
If you prefer to initialize it in code instead, you could modify the script like this:
Offline Mode Documentation shows this pattern of using [SerializeField]
for the Realtime reference in their examples.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@412870643533479936> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
@kapa.ai im loss
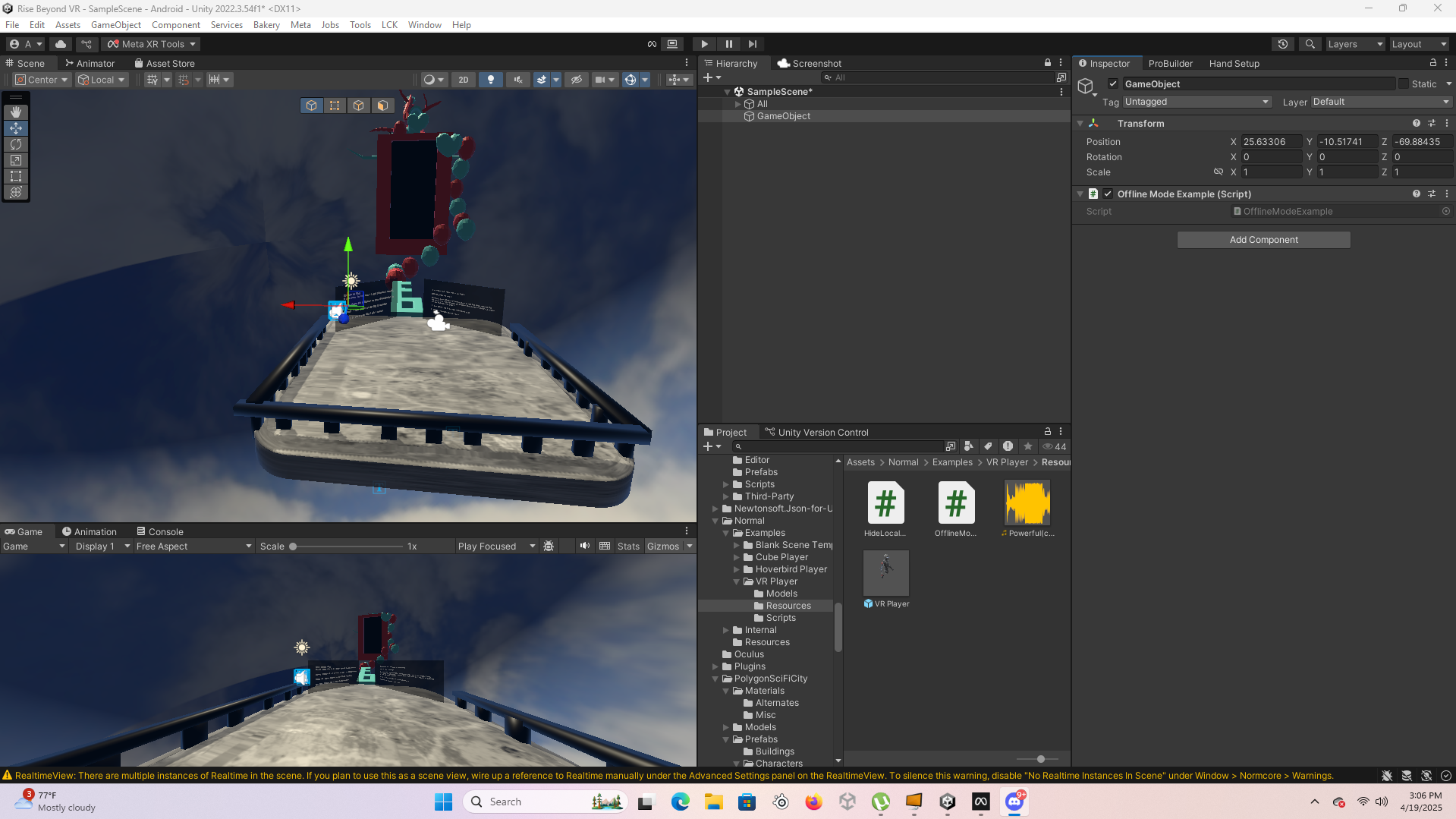
Let me check the knowledge base for you again. One moment! :books:
----------------------
I understand that you might be feeling lost with the Normcore setup we've been discussing. Let me simplify things for you:
Simple Steps to Set Up Offline/Online Switching
1. For your offline rig (which you already have):
- This is your local player representation when not connected to a server
2. For Normcore connection:
- Add a GameObject with a Realtime component to your scene
- Add a RealtimeAvatarManager to the same GameObject
- Set your App Key in the Realtime component
3. For your networked avatar:
- Create a prefab with RealtimeView and RealtimeAvatar components
- Put it in a Resources folder
- Set this as the "Local Avatar Prefab" in the RealtimeAvatarManager
4. For switching between them:
- Add the OfflineRigManager script we discussed
- Connect it to your offline rig and Realtime component
If you'd like to start with a working example, I recommend checking out the XR Avatars and Voice Chat guide which provides a complete walkthrough with sample projects.
Would it help if I broke down any specific part of this process in more detail?
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------
<@1245530684240695310> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
i just want it to disabe a thing i pcik when a server is conted @kapa.ai
Let me check the knowledge base for you again. One moment! :books:
----------------------
I understand you want to disable a specific object when connecting to a Normcore server. Let's simplify this:
Based on our previous conversation, you can use the
didConnectToRoom
event from the Realtime component to disable any GameObject when a connection is established. Here's a simple script to do exactly that:
To set this up:
1. Add this script to any GameObject in your scene
2. In the Inspector, drag your Realtime component to the "realtime" field
3. Drag the GameObject you want to disable to the "objectToDisable" field
That's it! When you connect to a Normcore room, your selected object will be disabled automatically, and when you disconnect, it will be enabled again.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1245530684240695310> Kapa.ai is still learning and improving, please let me know how I did by reacting below.
the error message says you have multiple Realtime in the scene and have to specify which one you are using. Easiest fix is to remove the other Realtime.
k