How to disable and enable the prefab with normcore
Hi. I'm new to the multiplayer game.
I'd like to disable and enable the prefab in my game while running.
I'm using .SetActive() now.
How do I use normcore?
Thank you in advance for the answer.
Solution:Jump to solution
----------------------
If you want to remove the object at index 0 after finishing the loop, you can do so by calling the
RemoveAt()
method on the list. Here's how you can modify the previous example:
```csharp
using UnityEngine;...54 Replies
Hi @korimeily. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
In Normcore, you can use Realtime prefabs which are synchronized via the Realtime API using realtime views and realtime components. To disable and enable a prefab, you would typically use the
SetActive()
method in Unity. However, in a multiplayer setting with Normcore, you need to ensure that this change is synchronized across all clients.
To do this, you can use the Realtime API's ownership and lifetime flags. These flags give you control over which clients can modify data in your rooms. For example, you can set a flag to denote that a certain client has the authority to enable or disable a prefab.
Here's a basic example of how you might use these flags:
In this example, realtimeView
is a reference to the RealtimeView
component on your prefab. This component is what allows Normcore to synchronize the prefab across all clients. The isOwnedLocally
property is a boolean that tells you whether the current client is the owner of the prefab.
Remember, any changes you make to a prefab should be done through the Realtime API to ensure they are properly synchronized. For more detailed information, you can refer to the Realtime API documentation (https://normcore.io/documentation/realtime).
Please note that this is a simplified example and your actual implementation may need to be more complex depending on your game's specific requirements.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@455563652175495174> kapa.ai is still learning and improving, please let us know how it did by reacting below
This property is obsoleted. @Normcore Assistant
Let me check the knowledge base for you. One moment! :books:
----------------------
I apologize for the confusion. If the
isOwnedLocally
property is obsolete, you can use the isOwnedLocallySelf
or isOwnedLocallyInHierarchy
properties instead. These properties are part of the RealtimeView
and RealtimeComponent
classes in Normcore.
isOwnedLocallySelf
is a boolean that specifies whether the component is owned by the local client. isOwnedLocallyInHierarchy
is a boolean that specifies whether the component or any of its parent views are owned by the local client.
Here's how you might use these properties:
In this example, realtimeView
is a reference to the RealtimeView
component on your prefab. This component is what allows Normcore to synchronize the prefab across all clients.
For more detailed information, you can refer to the RealtimeView documentation (https://normcore.io/documentation/reference/classes/Normal.Realtime.RealtimeView) and the RealtimeComponent documentation (https://normcore.io/documentation/reference/classes/Normal.Realtime.RealtimeComponent%601).
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@455563652175495174> kapa.ai is still learning and improving, please let us know how it did by reacting below
I have a list that contains prefabs and I'd like to enable each prefab when I click the button.
@Normcore Assistant
Let me check the knowledge base for you. One moment! :books:
Sorry @korimeily, I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?
easiest method is to make a custom model with a bool for set active. when the model changed event fires you set the game object active/ inactive. but for the view to be registered it needs to instantiate active so it might be better to set a child object as inactive if you need it to start hidden
@Normcore Assistant how do i make a custom model with a bool property called setActive
Let me check the knowledge base for you. One moment! :books:
----------------------
To create a custom model with a bool property called
setActive
in Normcore, you would first define a class with the [RealtimeModel]
attribute. Inside this class, you would define a private bool field with the [RealtimeProperty]
attribute. Here's an example:
In this example, CustomModel
is the name of your custom model. _setActive
is the private field that will hold the value of your setActive
property. The RealtimeProperty
attribute tells Normcore to synchronize this field across all clients. The first argument to RealtimeProperty
is the property ID, which must be unique within this model. The second argument specifies whether the property is reliable (i.e., whether changes to the property should always be sent to the server, even if some packets are lost).
After defining your model, you would need to compile it. If you're using Unity 2019 or 2020, you can do this by going back to Unity, highlighting the CustomModel.cs
file in your project, and clicking the "Compile Model" button in the inspector. If you're using Unity 2021 or newer, Normcore will automatically generate the remaining code needed to use your model when your project compiles.
For more detailed information, you can refer to the Creating a custom realtime component (https://normcore.io/documentation/realtime/synchronizing-custom-data) and Creating a realtime model (https://normcore.io/documentation/realtime/synchronizing-custom-data#creating-a-realtime-model) sections of the Normcore documentation.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@162940911964258305> kapa.ai is still learning and improving, please let us know how it did by reacting below
@Normcore Assistant how do i use that model to set my gameobject active when the model changes
Let me check the knowledge base for you. One moment! :books:
----------------------
To use the custom model to set your GameObject active when the model changes, you would need to create a
RealtimeComponent
subclass that uses your custom model. In this subclass, you would override the OnRealtimeModelReplaced()
method to subscribe to changes to the setActive
property. Here's an example:
In this example, CustomComponent
is the name of your custom component. OnRealtimeModelReplaced()
is a method that is called when the model for this component is replaced (e.g., when the component is first created or when switching rooms). setActiveDidChange
is an event that is fired when the setActive
property on the model changes.
You would attach this CustomComponent
script to the GameObject that you want to set active or inactive based on the setActive
property of the model.
For more detailed information, you can refer to the Creating a custom realtime component (https://normcore.io/documentation/realtime/synchronizing-custom-data) and Creating a realtime model (https://normcore.io/documentation/realtime/synchronizing-custom-data#creating-a-realtime-model) sections of the Normcore documentation.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@162940911964258305> kapa.ai is still learning and improving, please let us know how it did by reacting below
I have a list that contains prefabs and I'd like to enable each prefab when I click the button. and when prefabs are active another player can see @Normcore Assistant
Let me check the knowledge base for you. One moment! :books:
----------------------
To enable each prefab in a list when a button is clicked and ensure that other players can see the changes, you can use Normcore's
Realtime.Instantiate()
method. This method instantiates a prefab and synchronizes it across all clients.
Here's a basic example of how you might do this:
In this example, _realtime
is a reference to the Realtime
component in your scene, _prefabs
is a list of the prefabs you want to enable, and _button
is the button that will enable the prefabs when clicked. The EnablePrefabs()
method is called when the button is clicked, and it iterates over each prefab in the list, instantiates it using Realtime.Instantiate()
, and enables it using SetActive(true)
.
Remember to add the prefabs to a Resources folder in your Unity project, as Normcore requires prefabs to be in a Resources folder to instantiate them at runtime.
For more detailed information, you can refer to the Creating a Player Controller (https://normcore.io/documentation/guides/creating-a-player-controller#making-it-multiplayer) and Creating a prefab (https://normcore.io/documentation/realtime#prefabs) sections of the Normcore documentation.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@455563652175495174> kapa.ai is still learning and improving, please let us know how it did by reacting below
I use Onclick() event button. How do I use it? @Normcore Assistant
Let me check the knowledge base for you. One moment! :books:
----------------------
To use the
OnClick()
event of a button in Unity, you need to add a method to the button's onClick
event. This method will be called whenever the button is clicked. Here's a basic example:
In this example, _button
is a reference to the Button
component on your button. The ButtonClicked
method is added to the button's onClick
event in the Start
method. This means that the ButtonClicked
method will be called whenever the button is clicked.
You can replace the Debug.Log
call in the ButtonClicked
method with whatever code you want to execute when the button is clicked.
For more detailed information, you can refer to the Button.onClick documentation (https://docs.unity3d.com/ScriptReference/UI.Button-onClick.html) in the Unity documentation.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@455563652175495174> kapa.ai is still learning and improving, please let us know how it did by reacting below
I've already have my prefabs in the scene game but setfalse. I'd like to set active each prefab when click the button then it setactive. How do I synchronizes?
@Normcore Assistant
Let me check the knowledge base for you. One moment! :books:
Sorry @korimeily, I seem to be having a bit of trouble. Don't worry though, I've pinged my support and they will try to fix me up! :technologist:
Perhaps try again in 15mins?
I've already have my prefabs in the scene game but setfalse. I'd like to set active each prefab when click the button then it setactive. How do I synchronizes?
@Normcore Assistant
you add a realtime view to the parent of each prefab (it wont work on the disabled object itself as it needs to be active to register with realtime) then you set the value of the custom model i posted earlier to true when you click your ui button. e.g. model.setActive = true;
if you follow the custom model tutorial it will make more sense
I following this code and there's no "Compile Model" button in the inspector, pls help.
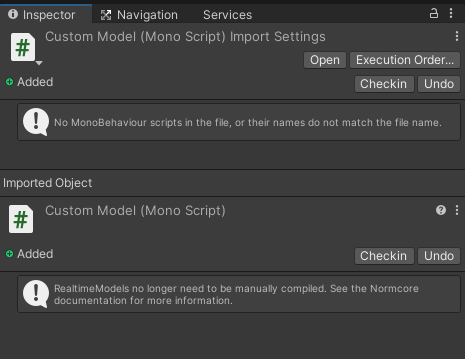
@Mechabit
that's only in the old version, in the newer version there's no compile button
it says so in the text on the bottom
How should I do? @Mechabit
it's already compiled, go to the next step
make a realtime component script
thank you very much🥹
I follow the steps but it doesn't work🥹 🥹 My parent obj is inactive when I run the program and another player can't see the child obj.
public class CustomComponent : RealtimeComponent<CustomModel>
{
public List<GameObject> shark = new List<GameObject>();
public void Shark()
{
for (var i = 0; i < shark.Count; i++)
{
shark[0].SetActive(true);
break;
}
shark.RemoveAt(0);
}
protected override void OnRealtimeModelReplaced(CustomModel previousModel, CustomModel currentModel)
{
if (previousModel != null)
{
// Unsubscribe from events on the previous model
previousModel.setActiveDidChange -= SetActiveDidChange;
}
if (currentModel != null)
{
// Subscribe to events on the new model
currentModel.setActiveDidChange += SetActiveDidChange;
// Set the initial active state of the GameObject
gameObject.SetActive(currentModel.setActive);
}
}
private void SetActiveDidChange(CustomModel model, bool value)
{
// Update the active state of the GameObject when the model changes
gameObject.SetActive(true);
}
here is my code. If I made a mistake somewhere, please tell me.
@Mechabit
I've got no idea what you're trying to do here
you need each shark to have a separate parent with the custom component on it and you need to set the child object to inactive, not the parent
like this
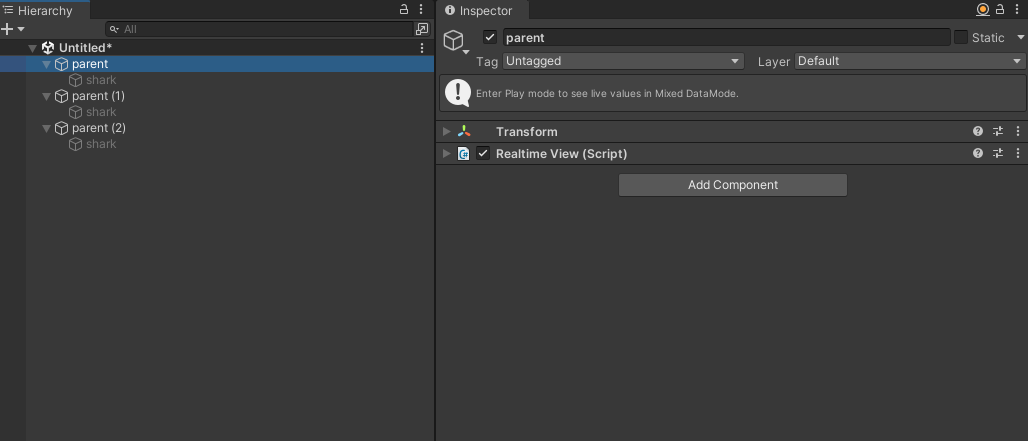
then you set the child active when the model changes
I set it up like this initially. I want the shark objects in the list to come out one at a time if I press the UI button. and another player can see the changes. what should I do with the custom component?
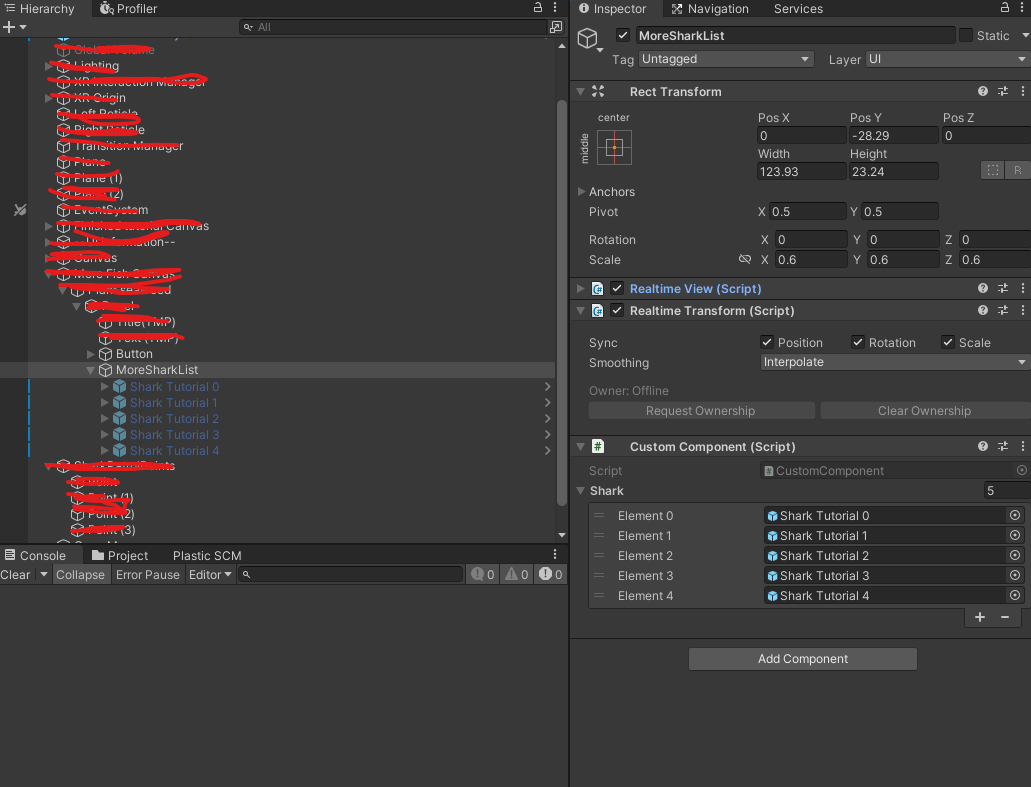
@Mechabit
maybe there's an easier method that doesn't need custom models
instead of deactivating the objects you can set the scale to 0,0,0
put a realtime transform on each shark
that will sync the scale of each shark
then when you press your button you scale the shark up to 1,1,1
you also need ownership of the realtime transform
like this
GetComponent<RealtimeTransform>().RequestOwnership();
transform.localScale = new Vector3(1,1,1);
Sorry for my bad English. I want the shark objects in the list(each element) to come out if click the UI button ex. when click the button it will setactive shark obj(element0). How should I do with the RequestOwnership? @Mechabit
request ownership just tells normcore which client is in control of the object
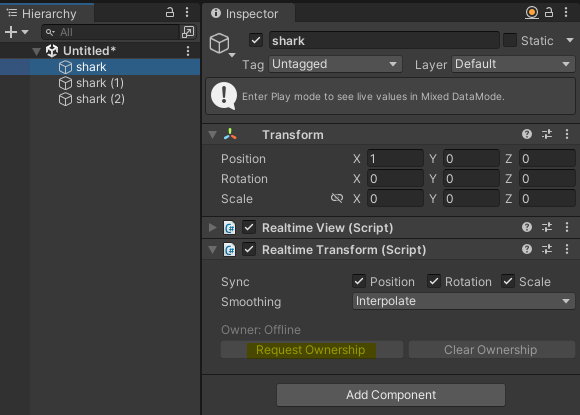
there's a button in the inspector for you to test what happens when you request ownership
the easiest way to set realtime transform and realtime view in object in the list @Normcore Assistant
Let me check the knowledge base for you. One moment! :books:
----------------------
To set
RealtimeTransform
and RealtimeView
to objects in a list, you can iterate over the list and add these components to each object. Here's a basic example:
In this example, _objects
is a list of the objects you want to add RealtimeTransform
and RealtimeView
to. The Start()
method is called when the script is first run, and it iterates over each object in the list, adding RealtimeTransform
and RealtimeView
to each one.
Remember, RealtimeTransform
and RealtimeView
are components provided by Normcore that allow you to synchronize a GameObject's transform and state across all clients in a multiplayer setting. For more detailed information, you can refer to the RealtimeTransform (https://normcore.io/documentation/realtime/realtimetransform) and RealtimeView (https://normcore.io/documentation/realtime/realtimeview) sections of the Normcore documentation.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@455563652175495174> kapa.ai is still learning and improving, please let us know how it did by reacting below
@Normcore Assistant not add component but set request ownership
Let me check the knowledge base for you. One moment! :books:
----------------------
To request ownership of
RealtimeTransform
and RealtimeView
components for each object in a list, you can iterate over the list and call RequestOwnership()
on each component. Here's a basic example:
In this example, _objects
is a list of the objects you want to request ownership of. The Start()
method is called when the script is first run, and it iterates over each object in the list, requesting ownership of the RealtimeTransform
and RealtimeView
components on each one.
Remember, RequestOwnership()
is a method provided by Normcore that allows a client to request ownership of a RealtimeComponent
or RealtimeModel
. Ownership is a concept in Normcore that determines which client has the authority to make changes to a component or model. For more detailed information, you can refer to the Ownership and Lifetime Flags (https://normcore.io/documentation/room/ownership-and-lifetime-flags) section of the Normcore documentation.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@455563652175495174> kapa.ai is still learning and improving, please let us know how it did by reacting below
change for each to for, please? @Normcore Assistant
Let me check the knowledge base for you. One moment! :books:
----------------------
Sure, you can use a
for
loop instead of a foreach
loop to iterate over the list. Here's how you can modify the previous example:
In this example, i
is the index of the current object in the list. _objects[i]
gets the object at the current index. The rest of the code is the same as in the previous example.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@455563652175495174> kapa.ai is still learning and improving, please let us know how it did by reacting below
add remove index at 0 after finished loop @Normcore Assistant
Let me check the knowledge base for you. One moment! :books:
Solution
----------------------
If you want to remove the object at index 0 after finishing the loop, you can do so by calling the
RemoveAt()
method on the list. Here's how you can modify the previous example:
In this example, RemoveAt(0)
is called on _objects
after the for
loop. This removes the object at index 0 from the list. The if (_objects.Count > 0)
check is there to ensure that there is at least one object in the list before trying to remove one.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@455563652175495174> kapa.ai is still learning and improving, please let us know how it did by reacting below
@Tom why's this bot adding double braces in this code?
:jumpblown: crazy! ill report that now