15 Replies
Hi @Trần Tiến Đạt. I'm kapa.ai, your friendly support bot. I will try to answer your question by searching through the knowledge base. :wave:
----------------------
To synchronize a Color Array in NormalVR, you would need to create a custom RealtimeComponent and a corresponding RealtimeModel. The process is similar to synchronizing a single color, but you would need to handle an array of colors instead.
Here's a step-by-step guide based on the information provided in the knowledge sources:
1. Create a RealtimeModel: Start by creating a
ColorSyncModel.cs
script. This model will hold the array of colors you want to synchronize. The model should be a partial
class and should not be a subclass of any other class. You would need to add a [RealtimeModel]
attribute to the class and a [RealtimeProperty]
attribute to the color array variable. The color array variable should be private and start with an underscore.
2. Generate Model Code: If you're using Unity 2019/2020, you will need to manually compile the model. Highlight the ColorSyncModel.cs
file in your project and click the “Compile Model” button in the inspector. Normcore will generate the necessary code to keep the model in sync with the datastore.
3. Create a RealtimeComponent: Create a ColorSync.cs
script that inherits from RealtimeComponent<ColorSyncModel>
. This component will handle the synchronization of the color array. You would need to implement methods to update the color array on the model and to handle changes to the color array.
4. Test the Component: Add the ColorSync
component to a game object in your Unity scene and test it out. You should see the colors of the game object update in real-time.
Please note that this is a simplified example and you may need to adjust the code to fit your specific needs. For more detailed information, you can refer to the Synchronizing Custom Data (https://normcore.io/documentation/realtime/synchronizing-custom-data) guide in the Normcore documentation.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@1152048129353461810> kapa.ai is still learning and improving, please let us know how it did by reacting below
I tried and got this error
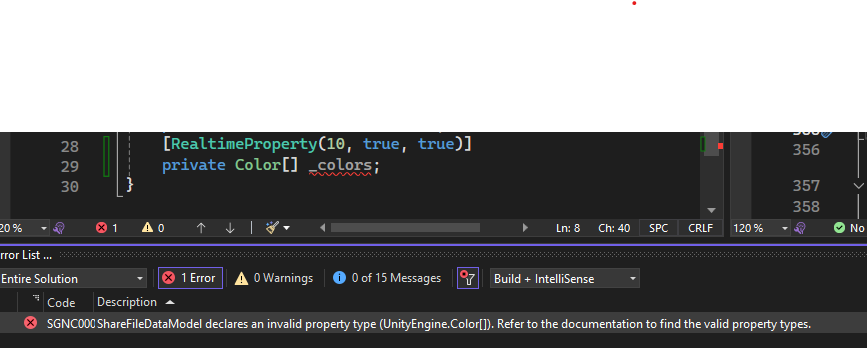
what you actually want is a realtime collection
Gist
How to use RealtimeDictionary
How to use RealtimeDictionary. GitHub Gist: instantly share code, notes, and snippets.
store a dictionary of models containing a single color property
@Normcore Assistant how do I use a realtime dictionary to sync an array of colors
Let me check the knowledge base for you. One moment! :books:
----------------------
To synchronize an array of colors using a RealtimeDictionary in NormalVR, you would need to create a custom RealtimeComponent and a corresponding RealtimeModel. The process is similar to synchronizing a single color, but you would need to handle a dictionary of colors instead.
Here's a step-by-step guide based on the information provided in the knowledge sources:
1. Create a RealtimeModel: Start by creating a
ColorSyncModel.cs
script. This model will hold the dictionary of colors you want to synchronize. The model should be a partial
class and should not be a subclass of any other class. You would need to add a [RealtimeModel]
attribute to the class and a [RealtimeProperty]
attribute to the color dictionary variable. The color dictionary variable should be private and start with an underscore.
2. Generate Model Code: If you're using Unity 2019/2020, you will need to manually compile the model. Highlight the ColorSyncModel.cs
file in your project and click the “Compile Model” button in the inspector. Normcore will generate the necessary code to keep the model in sync with the datastore.
3. Create a RealtimeComponent: Create a ColorSync.cs
script that inherits from RealtimeComponent<ColorSyncModel>
. This component will handle the synchronization of the color dictionary. You would need to implement methods to update the color dictionary on the model and to handle changes to the color dictionary.
4. Test the Component: Add the ColorSync
component to a game object in your Unity scene and test it out. You should see the colors of the game object update in real-time.
Please note that this is a simplified example and you may need to adjust the code to fit your specific needs. For more detailed information, you can refer to the Synchronizing Custom Data (https://normcore.io/documentation/realtime/synchronizing-custom-data) guide in the Normcore documentation.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@162940911964258305> kapa.ai is still learning and improving, please let us know how it did by reacting below
bad bot, go train more
@Normcore Assistant how do I use a RealtimeDictionary to sync a model with a color property?
Let me check the knowledge base for you. One moment! :books:
----------------------
To synchronize a model with a color property using a RealtimeDictionary in NormalVR, you would need to create a custom RealtimeComponent and a corresponding RealtimeModel. The process is similar to synchronizing a single color, but you would need to handle a dictionary of colors instead.
Here's a step-by-step guide based on the information provided in the knowledge sources:
1. Create a RealtimeModel: Start by creating a
ColorSyncModel.cs
script. This model will hold the dictionary of colors you want to synchronize. The model should be a partial
class and should not be a subclass of any other class. You would need to add a [RealtimeModel]
attribute to the class and a [RealtimeProperty]
attribute to the color dictionary variable. The color dictionary variable should be private and start with an underscore.
2. Generate Model Code: If you're using Unity 2019/2020, you will need to manually compile the model. Highlight the ColorSyncModel.cs
file in your project and click the “Compile Model” button in the inspector. Normcore will generate the necessary code to keep the model in sync with the datastore.
3. Create a RealtimeComponent: Create a ColorSync.cs
script that inherits from RealtimeComponent<ColorSyncModel>
. This component will handle the synchronization of the color dictionary. You would need to implement methods to update the color dictionary on the model and to handle changes to the color dictionary.
4. Test the Component: Add the ColorSync
component to a game object in your Unity scene and test it out. You should see the color of the game object update in real-time.
Please note that this is a simplified example and you may need to adjust the code to fit your specific needs. For more detailed information, you can refer to the Synchronizing Custom Data (https://normcore.io/documentation/realtime/synchronizing-custom-data) guide in the Normcore documentation.
----------------------
Note: you can ask me a follow up question by @ mentioning me again :speech_balloon:
----------------------<@162940911964258305> kapa.ai is still learning and improving, please let us know how it did by reacting below
guess this bot doesn't know the answer
I'm having trouble converting texture2d into a byte array and then using it for synchronization. I tried using byte[] and it was quite slow, so I'm thinking about using RealtimeArray, will it be better? Or another way is to convert Texture2D into a color array and synchronize with RealtimeArray or RealtimeDictionary<ColorModel> ?
syncing a texture that way will be slow even with a 32x32 resolution
might be better off uploading it to amazon cloud
oh I understand, thank you